Abstract
This blog post documents the creation of a simple “web-app” for viewing articles from simple.wikipedia and en.wikipedia side-by-side, and expounds on the importance of a “do-it-yourself”-attitude and a “minimalistic-mindset” to the programmer.
The finished product described here can be seen at the following URL:
https://kundalinisoftware.com/io/wikicompare.html
Form Follows Function
The phrase “form follows function” comes from architecture; it is the idea that the shape of a building should be determined mostly by its intended use — not by purely aesthetic concerns.
This principle often serves us well when programming, especially in today’s world of user-interface-centric software, where sparkly and over-engineered user interfaces often just create unneccessary layers between the user and the work they are trying to accomplish.
So with the principle of form following function — mixed with a healthy dose of minimalism — in mind, in this post we will go through creating a simple & ugly, but useful, utility.
Simple Wikipedia
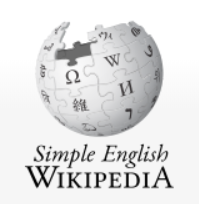
I found out recently about “simple wikipedia”, which is a version of wikipedia written for non-native speakers of English, or those who could otherwise benefit from articles written in a simpler style.
One interesting thing about wikipedia is that it exists in many languages.
A quick look at the URLs in use for the articles bear this out.
For example, here is the English wikipedia article about my home state, Ohio:
https://en.wikipedia.org/wiki/Ohio
And here is the same article, but in the German edition of Wikipedia:
https://de.wikipedia.org/wiki/Ohio
As you can see, there is a two letter prefix in each URL that identifies the language: EN for english, and DE for German.
Interestingly, the prefix simple can be used instead, to pull up the “simple English” version of the entry:
https://simple.wikipedia.org/wiki/Ohio
Personally, I think wikipedia is the most amazing thing ever, and I find myself consulting it all the time. But one frustration I have encountered when reading articles is that they are too hard, and contain too much detail!
After all, I am a layman, and often just need a simple explanation of a subject.
Enter: simple wikipedia!
Additionally, recently I had the idea that it would be nice to view simple wikipedia articles side-by-side with the standard english wikipedia articles.
As an aside, I have to admit that ideas come to me all the time, and for the most part, after my initial excitement, I just end up forgetting about them without ever actually implementing my ideas at all.
Tangent: Yogic-Philosophy

A quick aside here. One (non-western) paradigm I really like is the concept of the chakra system. In Hindu or Yogic philosophy, the chakras are thought to be energy centers in the body that represent different facets or characteristics of consciousness. The crown chakra is conceptualized of existing at the top of the head, and connects us to the source of our inspiration and limitless ideas. Proceeding downwards, eventually we reach the root chakra, which is more associated with material existence, and survival-oriented concerns — it is in essence the force that keeps us anchored into material reality!
Under ideal conditions, all the differing aspects of consciousness will be fully present in a person, and the person will be functioning at a peek level. Alas, however, this is normally not the case! In me, for example, traditionally I was overly active in the crown chakra, but very weak in the root chakra. This caused me to be ungrounded and impractical, and lost in my own head. (I eventually become more balanced when I started to work more on developing my body, such as getting into running and yoga asana.)
So to counter-act this tendency, recently I have vowed to try to implement — if if that just means creating prototypes — as many of my ideas as I can.
K.I.S.S.
To do this, one programming philosophy I have started to adopt is the KISS principle: Keep It Simple Stupid! To me, this phrase means that often the simple approach is just fine for our needs, and there is no need to overly complicate things.
Plus, I have found that building a prototype of something is often a fun way to learn how to do something, and is much more of an effective learning strategy than simply reading about a topic. Additionally, often the prototype itself is enough: the functionality that it implements is enough to suit our purposes. Plus if we do ever decide to make a fancier or more functional version, our prototype becomes the base upon which to build.
With those thoughts in mind, I set out today to build a simple HTML “web app” that accomplishes my goal of loading up an article from both the regular english wikipedia and simple wikipedia, and displays them side-by-side. (An even though now-a-days the concept of a “web-app” has achieved a ridiculous level of DOM-manipulating sophistication, for our purposes here, a web-app is simply an online web-page that implements some level of user-interactivity.)
When writing these blog posts, part of my intention is to convey a little bit about how my thought process goes, or in other words, how I go about creating these projects. Obviously, I also spend some time going through how the finished product works, but I think even more important than that is to attempt to show how to go about the process of programming.
Meet the IFRAME
One amazing fact about web pages is that they can contain within them other complete web pages. This is accomplished by using the HTML tag iframe, which stands for inline frame.
So as soon as my idea to display the two wikipedia articles side-by-side, I immediately thought of iframes. Additionally, before I even wrote any code at all, I googled the phrase: “iframe side by side” and started to look for code examples of how to use CSS to align two iframes side-by-side.
Within several minutes, I had the HTML I needed, complete with inline CSS:
<iframe onload="setupLinks();" id='frame1' src="" frameborder="0" scrolling="yes" style="height: 100%; width: 49%; float: left; " height="100%" width="49%" align="left"</iframe> <iframe onload="setupLinks();" id='frame2' src="" frameborder="0" scrolling="yes" style="overflow: hidden; height: 100%; width: 49%;" height="100%" width="49%" align="right"></iframe>
Next, I added a little text box to type in, and a button to click to update the screen:
<input type="text" id="SearchText" value=""> <button onclick="SetupFrames('');">Compare</button>
Finally, I whipped up a few lines of simple Javascript:
function SetupInitialFrame() { var urlParams = new URLSearchParams(window.location.search); // if we were invoked with a query parameter, defualt to using it... // this is so we can link to this page like this: // wikicompare.html?q=Football subject = urlParams.get('q'); // otherwise just default to showing them something hard, so they can appreciate the // simplicity of the simple.wikipedia article if (subject == '') subject = 'Quantum Mechanics'; SetupFrames(subject); } function SetupFrames(theText) { if (theText == '') searchText = document.getElementById("SearchText").value; else searchText = theText; frame1 = document.getElementById("frame1"); frame1.src="https://simple.wikipedia.org/w/index.php?title=" + searchText; frame2 = document.getElementById("frame2"); frame2.src="https://en.wikipedia.org/w/index.php?title=" + searchText; } function zoomLeft() { searchText = document.getElementById("SearchText").value; window.location.href = "https://simple.wikipedia.org/w/index.php?title=" + searchText; } function zoomRight() { searchText = document.getElementById("SearchText").value; window.location.href = "https://en.wikipedia.org/w/index.php?title=" + searchText; }
As you can see, there are only 4 functions. SetupInitialFrame() is called on onload, and is responsible for grabbing the (optional) query parameter the script was called with.
For example, if our script is invoked like this:
wikicompare.html?q=Football
then SetupInitialFrame() will call SetupFrames(‘Football).
Otherwise SetupInitialFrame() will just default to passing in the string ‘Quantum Mechanics‘ — since after-all what subject could be better served by a simplified article!
SetupFrames() is also called when the user clicks the search button; when the buttons are clicked, no string is passed in, so the function just graphs the value from the text box.
Finally, the functions zoomLeft() and zoomRight() just use window.location.href to go to full-page versions of either the simple or standard English version of the article, respectfully.
And that’s it: simple, ugly, and useful, all rolled into one!
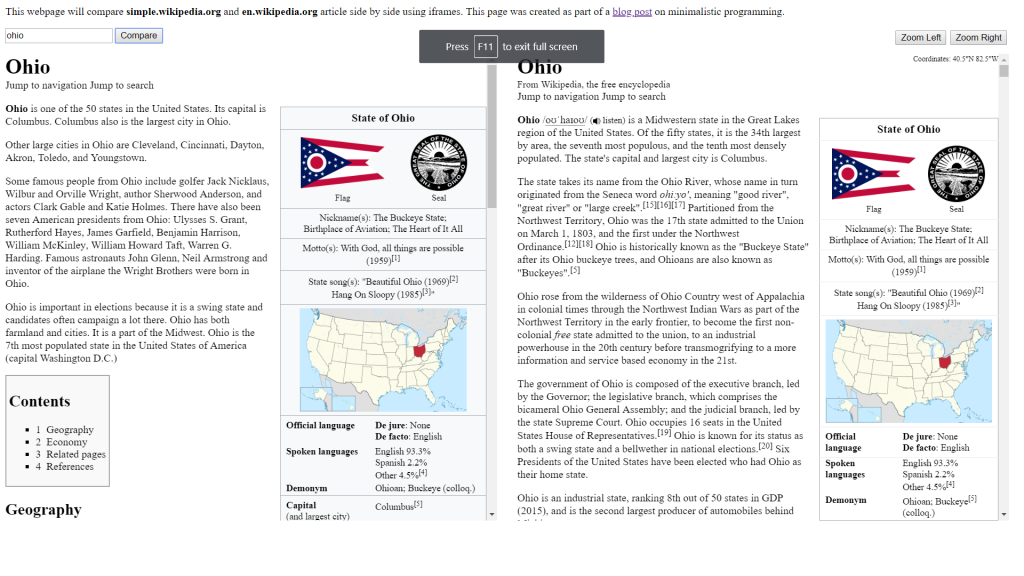
Conclusion
This post has documented the process of creating a simple and straight-forward web app that performs a useful function.
Learning to implement quick-and-dirty version of our ideas — using the resources, tools & programming knowledge that we have at our disposal to do so — is a vital skill for everyone to learn.
I say this because programming is the modern version of literacy, and people who don’t know how to do it are at a definite disadvantage.
Therefore, everyone should be encouraged to adopt the “hacker mindset” — a mindset that views computers not as things to be endlessly stared at, but as tools for building new things.
It is with the adoption of this mindset that we are able to become the empowered co-creaters of our world that we are meant to be.
Next Steps
- Get out there and learn to program!
- Checkout this followup post, which utilizes some of the ideas here, but from within the context of Python, TamperMonkey scripts, and the Google App Engine.
- Visit the Github repository for this code